3D Tilt Effect
The 3D Tilt Effect is an interactive visual technique that makes cards or images respond to mouse movements, creating a perspective effect. When a user moves their cursor over the card, it appears to tilt as if it were a 3D object. This effect is achieved through mouse tracking and perspective transform techniques, allowing the card to subtly bend in response to the user’s actions.
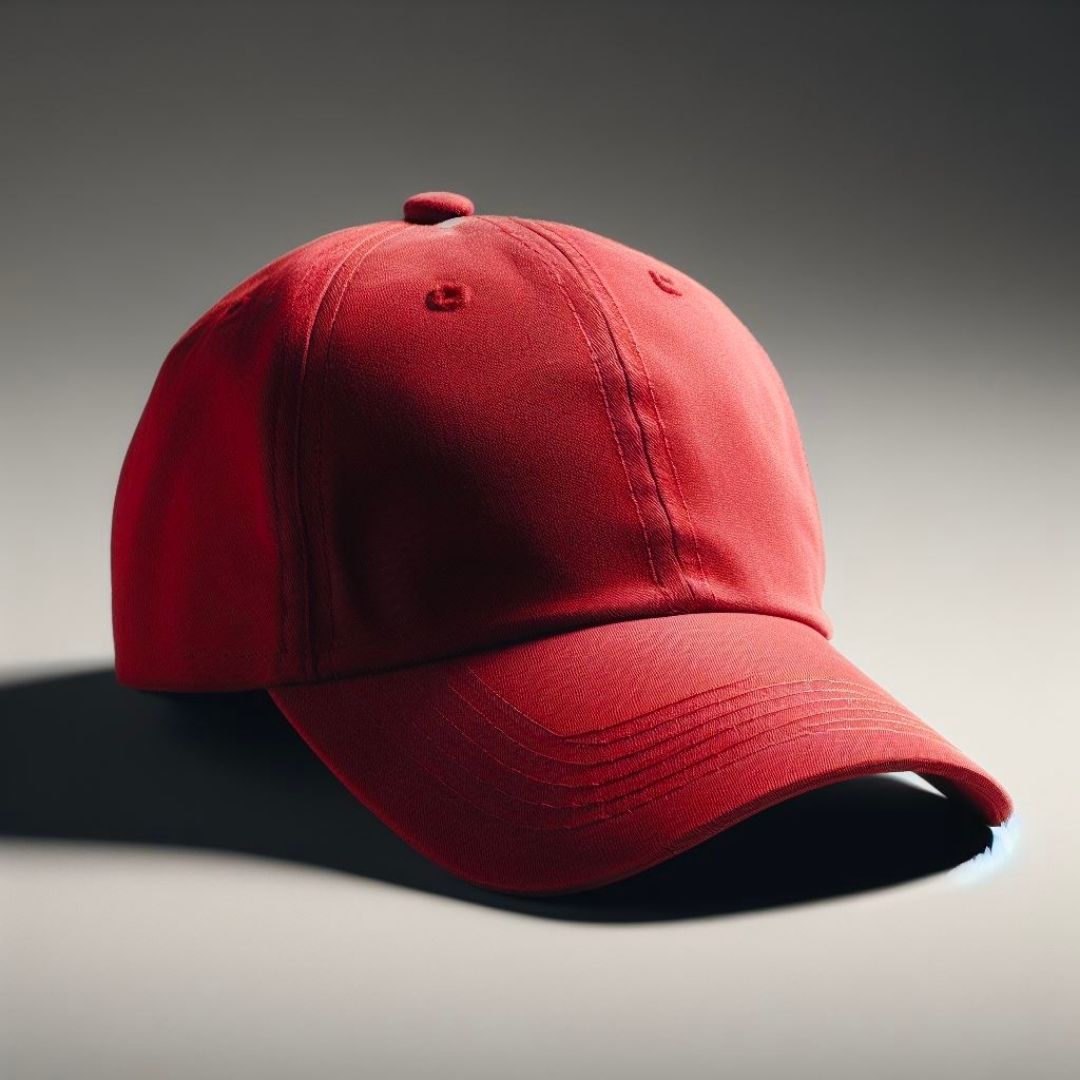
Red Hat
Best Sellers
Installation
Install the following dependencies:
npm i motion clsx tailwind-merge
Add util file
import { clsx, type ClassValue } from "clsx"
import { twMerge } from "tailwind-merge"
export function cn(...inputs: ClassValue[]) {
return twMerge(clsx(inputs))
}
Copy and paste the following code into your project.
import { Three3DTiltEffect } from "@/components/core/3d-tilt-effect";
import Image from "next/image";
import { Card, CardContent } from "@/components/ui/card";
export default function ThreeDTiltEffectExample() {
return (
<Three3DTiltEffect>
<Card className="pt-0 overflow-hidden md:w-[280px]">
<Image
src="https://bundui-images.netlify.app/products/04.jpeg"
alt=""
width={200}
height={200}
className="w-full aspect-square object-cover transition-all duration-200 ease-linear size-full"
unoptimized
/>
<CardContent>
<div className="text-xl">Red Hat</div>
<div className="text-sm text-muted-foreground">Best Sellers</div>
</CardContent>
</Card>
</Three3DTiltEffect>
);
}
API
Prop | Type | Default | Description |
---|---|---|---|
children | ReactNode | The content to be wrapped with the tilt effect | |
tiltFactor | number | 12 | The intensity of the tilt effect (higher value = more tilt) |
perspective | number | 1000 | The perspective depth to simulate a 3D effect |
transitionDuration | number | 0.5 | The duration of the transition when tilting |